MPR¶
-
MLModule
¶ genre Resample
author MeVis Medical Solutions AG
package MeVisLab/Standard
dll MLResample1
definition MLResample1.def see also MPRPath
,Reformat
inherits from MPRLight
keywords multi
,planar
,reformat
,interaction
,oblique
Purpose¶
The module MPR
calculates a multi-planar reformatted image from its input image.
The reformatting requires sampling of the input image, which can be performed using different filters. The MPR is optimized (using fixed-point arithmetic) for int8 and int16 types but has a fallback implementation for other basic data types.
Depending on the Memory Access
mode, it uses different strategies to access the input image.
Usage¶
The MPR
outputs a reformatted slice (or a slice stack if the Size
parameter is != 1) that is defined by its center world position (Translation
) and a Rotation
.
The edge length of the reformatted output slices is given by the Field Of View
parameter in millimeters, the resolution is specified by the Output Size
parameter in pixels.
Example¶
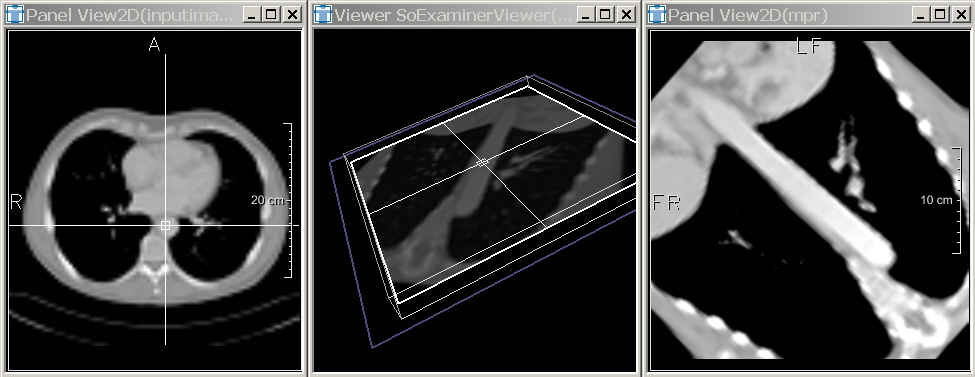
Details¶
The coordinate system of the output image is defined by the coordinate vectors wx, wy, and wz, which denote the direction of the image’s voxel rows, columns, and depth direction, respectively. These vectors are computed from the Rotation
parameter R by
(wx, wy, wz) = R (ex, ez, -ey),
where ex, ey, and ez are the unit vectors. This definition, especially the swapping of the y and z-direction, is compatible to the conventions used for Open Inventor draggers, which define the y-axis as the direction normal. Accordingly, the translation and rotation fields of an Inventor Dragger (e.g., SoJackDragger
, SoPlaneDragger
) can directly be connected to the fields of an MPR module.
In order to obtain the MPR rotation parameter for a given plane normal, compute:
rotation = SbRotation(SbVec3f(0, 1, 0), planeNormal);
If three points p1, p2 and p3 on a plane are given, the plane normal can be computed using the cross product:
SbVec3f dir1 = p2 - p1;
SbVec3f dir2 = p3 - p1;
SbVec3f planeNormal = dir1.cross(dir2);
The voxel-to-world-matrix W of the MPR’s output image is computed from the rotation R and the translation T by
W = T R M S D,
where
- D describes the translation from image center to origin (-sx/2, -sy/2, 0),
- S describes the voxel size, and
- M rotates y to z and z to -y.
Here is the corresponding code snippet:
double voxelSize = fieldOfView / outputSize;
dir1 = rotation * vec3(1, 0, 0) * voxelSize;
dir2 = rotation * vec3(0, 0, 1) * voxelSize;
dir3 = rotation * vec3(0, -1, 0) * voxelSize;
vec3 trans = translation - outputSize/2*dir1 - outputSize/2*dir2 - 1/2*dir3;
toWorldMatrix = mat4(dir1, dir2, dir3, trans);
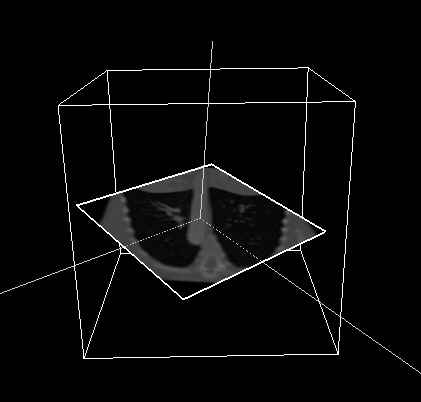
The above figure shows an input image box drawn in world coordinates and an MPR slice that defines an oblique slice within this box.
The center of the slice is given as the Translation
parameter, the slice normal is given by the Rotation
applied to the negative world z-axis.
Input Fields¶
input0¶
-
name:
input0
, type:
Image
¶ Input image from which an MPR is computed.
see also MPRLight.input0
Output Fields¶
output0¶
see also MPRLight.output0
Parameter Fields¶
Field Index¶
Visible Fields¶
Rotation¶
-
name:
rotation
, type:
Rotation
, default:
0 0 1 0
¶ Sets the rotation of the MPR slice, mapping the world X-, Y-, and Z-axes to the MPR image’s x-, negative z-, and y-axes, respectively.
The easiest way to edit the rotation is to use an Open Inventor dragger and connect its rotation field to this parameter.
Translation¶
-
name:
translation
, type:
Vector3
, default:
0 0 0
¶ Sets the translation vector to the center of the (first) MPR slice (in world coordinates).
Plane¶
-
name:
plane
, type:
Plane
, default:
0 0 1 0
¶ Sets the plane containing the first slice of the MPR output image.
Enable Plane Edit¶
-
name:
enablePlaneEdit
, type:
Bool
, default:
FALSE
¶ If checked, the
Plane
field can be used as an input field.Incremental modifications of the
Plane
field are transformed to modifications of theRotation
andTranslation
parameters.
Auto Center¶
-
name:
autoCenter
, type:
Bool
, default:
FALSE
¶ If checked, the
Translation
is internally moved so that the MPR is centered on the input image box.This is especially useful in combination with
Output Size Mode
== ‘Automatic’ and the “Slicing” tab.
Fill Value¶
-
name:
fillValue
, type:
Double
, default:
0
, deprecated name:
fillvalue
¶ Sets the value used for regions outside of the input image.
Output Size Mode¶
-
name:
outputSizeMode
, type:
Enum
, default:
AspectRatio
¶ Defines how the output size is defined.
Values:
Title | Name | Description |
---|---|---|
Aspect Ratio | AspectRatio | Uses a single size and an aspect ratio to define the output size. Only equal voxel sizes in x/y are possible. |
Independent | Independent | Allows for specifying the output size and field-of-view in x/y separately. |
Automatic | Automatic | Determines the output size automatically to match the input image size, taking the Rotation to account, so that orthogonal views along the input image have the same output size as a OrthoSwapFlip/Subimage combination would have. This mode should be used when using the “Slicing” tab. It is typically useful to also enable Auto Center . |
Field Of View¶
-
name:
fieldOfView
, type:
Double
, default:
100
¶ Sets the extent of the MPR slice in the world coordinate system in millimeters.
Output Size¶
-
name:
outputSize
, type:
Integer
, default:
256
, minimum:
1
¶ Sets the resolution of the output slice (x, y) in pixels.
Aspect Ratio¶
-
name:
aspectRatio
, type:
Double
, default:
1
¶ Sets the aspect ratio of the MPR.
Values larger than 1.0 produce portrait output, while values smaller than 1.0 produce landscape output.
Field Of View X¶
-
name:
fieldOfViewX
, type:
Double
, default:
100
¶ Sets the x-extent of the MPR slice in the world coordinate system in millimeters.
Field Of View Y¶
-
name:
fieldOfViewY
, type:
Double
, default:
100
¶ Sets the y-extent of the MPR slice in the world coordinate system in millimeters.
Output Size X¶
-
name:
outputSizeX
, type:
Integer
, default:
256
¶ Sets the x-resolution of the output slice in pixels.
Output Size Y¶
-
name:
outputSizeY
, type:
Integer
, default:
256
¶ Sets the y-resolution of the output slice in pixels.
Mode¶
-
name:
slabMode
, type:
Enum
, default:
SizeInSlicesForward
¶ Defines how the slab size is calculated.
Values:
Title | Name |
---|---|
Mm | SizeInMm |
Mm Forward | SizeInMmForward |
Slices | SizeInSlices |
Slices Forward | SizeInSlicesForward |
Slices Negative First | SizeInSlicesNegativeFirst |
Size¶
Size In Slices¶
Use Up Vector¶
Up Vector¶
-
name:
upVector
, type:
Vector3
, default:
0 1 0
¶ Sets an up vector that can be used to determine the orientation of the MPR axes within the plane.
If
Use Up Vector
is set, the MPR will be rotated around the center (Translation
) in order to align the y-axis with the up-vector.
Slice No.¶
-
name:
inputSliceNo
, type:
Integer
, default:
0
¶ Sets the slice number to be reproduced by the
Represent Input Slice
trigger.
Represent Input Slice¶
-
name:
representInputSlice
, type:
Trigger
¶ When pressed,
Field Of View
,Output Size
,Aspect Ratio
,Translation
, andRotation
are adjusted so that the output exactly matches the input slice numberSlice No.
.
Axial (axial)¶
-
name:
axial
, type:
Trigger
¶ When pressed, the MPR is set to axial (transversal) orientation in world coordinates.
Sagittal (sagittal)¶
-
name:
sagittal
, type:
Trigger
¶ When pressed, the MPR is set to sagittal orientation in world coordinates.
Coronal (coronal)¶
-
name:
coronal
, type:
Trigger
¶ When pressed, the MPR is set to coronal orientation in world coordinates.
Axial (axialOnInput)¶
-
name:
axialOnInput
, type:
Trigger
¶ When pressed, the MPR is set to axial (transversal) orientation on the input image.
It selects an orientation of the input image that is closest to the world axial orientation. The output of the MPR will be identical to that of an OrthoSwapFlip with axial settings.
Sagittal (sagittalOnInput)¶
-
name:
sagittalOnInput
, type:
Trigger
¶ When pressed, the MPR is set to sagittal orientation on the input image.
It selects an orientation of the input image that is closest to the world sagittal orientation. The output of the MPR will be identical to that of an OrthoSwapFlip with sagittal settings.
Coronal (coronalOnInput)¶
-
name:
coronalOnInput
, type:
Trigger
¶ When pressed, the MPR is set to coronal orientation on the input image.
It selects an orientation of the input image that is closest to the world coronal orientation. The output of the MPR will be identical to that of an OrthoSwapFlip with coronal settings.
Interpolation¶
-
name:
interpolation
, type:
Enum
, default:
Trilinear
, deprecated name:
Mode
¶ Defines the interpolation used for resampling.
Values:
Title | Name | Deprecated Name | Description |
---|---|---|---|
Nearest Neighbor | NearestNeighbor | Nearest Neigbour | No interpolation, picks the nearest input image voxel. Fastest mode. |
Trilinear | Trilinear | Trilinear(skip border),TrilinearSkipBorder | Trilinear filtering: The 8-voxel neighborhood is used for linear interpolation. Slowest mode. |
Border Handling¶
-
name:
borderHandling
, type:
Enum
, default:
UseFillValue
¶ Defines how borders are handled in trilinear filtering.
Values:
Title | Name | Description |
---|---|---|
Clamp To Edge | ClampToEdge | Clamps the interpolation to the edge of the input volume and uses the fill value only for values beyond the edge. ![]() |
Use Fill Value | UseFillValue | Uses the fill value for values on the border and outside of the volume. ![]() |
Memory Access¶
-
name:
memoryAccess
, type:
Enum
, default:
Global
, deprecated name:
pagedInputImage
¶ Defines how the module accesses the input image.
Values:
Title | Name | Deprecated Name | Description |
---|---|---|---|
Global | Global | FALSE,Paged,TRUE | Fastest, but highest memory consumption and initialization time. The input image is accessed as a global image (via the ML memory image). This consumes a lot of memory for large datasets. If not enough memory is available, the module falls back to the Virtual Volume memory access. |
Virtual Volume | VirtualVolume | Slowest, but works on huge datasets. All pages of the input image are requested via the virtual volume mechanism, thus only small portions of the input image are needed at the same time. |
Enable¶
-
name:
enableSlabProjection
, type:
Bool
, default:
FALSE
¶ If checked, the projection of the slab onto a single slice is enabled.
The
Depth Projection
andTime Projection
are used to define which projection method is used.
Depth Projection¶
-
name:
depthSlabRenderMode
, type:
Enum
, default:
Maximum
¶ Defines the render mode for depth projection.
Values:
Title | Name | Description |
---|---|---|
Minimum | Minimum | Minimum value is computed. |
Maximum | Maximum | Maximum value is computed. |
Average | Average | Average is computed. |
Keep Dimension | KeepDimension | The depth dimension is not projected, the output image will have the same number of slices as the input image. |
Time Projection¶
-
name:
timeSlabRenderMode
, type:
Enum
, default:
Maximum
¶ Defines the render mode for temporal projection.
Values:
Title | Name | Description |
---|---|---|
Minimum | Minimum | Minimum value is computed. |
Maximum | Maximum | Maximum value is computed. |
Average | Average | Average is computed. |
Keep Dimension | KeepDimension | The time dimension is not projected, the output image will have the same number of time points as the input image. |
Time Start¶
-
name:
timeSlabStart
, type:
Integer
, default:
0
¶ Sets the start time point for temporal projection.
Time Span¶
-
name:
timeSlabSize
, type:
Integer
, default:
1
¶ Sets the number of time points that are projected.
Enable Current Slice¶
-
name:
enableCurrentSlice
, type:
Bool
, default:
FALSE
¶ If checked, the
Current Slice
field and its synchronization is enabled.
Current Slice¶
Number Of Slices¶
Snap Current Slice To Integer If Orthogonal¶
-
name:
snapCurrentSliceToIntegerIfOrthogonal
, type:
Bool
, default:
TRUE
¶ If checked, the current slice is snapped to an integer when the rotation is orthogonal on the input image.
This allows the MPR to directly copy input voxels instead of using interpolation in orthogonal cases.
Image Color¶
-
name:
imageColor
, type:
Color
, default:
1 1 1
, deprecated name:
baseColor
¶ Sets the color used to display the image data.
Border Color¶
-
name:
borderColor
, type:
Color
, default:
1 1 1
¶ Sets the color used to render the slice border.
On (borderOn)¶
-
name:
borderOn
, type:
Bool
, default:
TRUE
¶ If checked, the rendering of the slice border is enabled.
On (drawImageOn)¶
-
name:
drawImageOn
, type:
Bool
, default:
TRUE
¶ If checked, the rendering of the image data is enabled.
Interactive manipulator¶
-
name:
manipulatorOn
, type:
Bool
, default:
TRUE
¶ If checked, a 3D manipulator that can be used to translate, rotate, and scale the MPR slice in the Open Inventor scene is enabled.
Activate clip plane¶
-
name:
clipPlaneOn
, type:
Bool
, default:
FALSE
¶ If checked, a clip plane applied to the Open Inventor scene on one side of the MPR plane is enabled.
Flip Clip Plane¶
-
name:
flipClipPlane
, type:
Bool
, default:
FALSE
¶ If checked, the half space that is clipped by the clip plane is flipped.
Clip Plane Offset¶
-
name:
clipPlaneOffset
, type:
Float
, default:
0
¶ Sets an offset to the clip plane in the direction of the MPR slice normal.
Draw axes¶
-
name:
axesOn
, type:
Bool
, default:
FALSE
, deprecated name:
drawVectors
¶ If checked, the rendering of the MPR output image coordinate axes is enabled.
Alpha¶
-
name:
alphaFactor
, type:
Float
, default:
1
, minimum:
0
, maximum:
1
¶ Sets an alpha value used to draw the image data.
Use Z buffer¶
-
name:
zBuffer
, type:
Bool
, default:
TRUE
¶ If disabled, the z-buffer is ignored when rendering the slice.
Blend Mode¶
-
name:
blendMode
, type:
Enum
, default:
BLEND_REPLACE
¶ Defines how the slice texture is rendered.
Values:
Title | Name |
---|---|
Replace | BLEND_REPLACE |
Screen | BLEND_SCREEN |
Add | BLEND_ADD |
Minimum | BLEND_MINIMUM |
Maximum | BLEND_MAXIMUM |
Reverse Subtract | BLEND_REVERSE_SUBTRACT |
Subtract | BLEND_SUBTRACT |
Blend | BLEND_BLEND |
Premultiplied Blend | BLEND_PREMULTIPLIED_BLEND |